My Doc Page
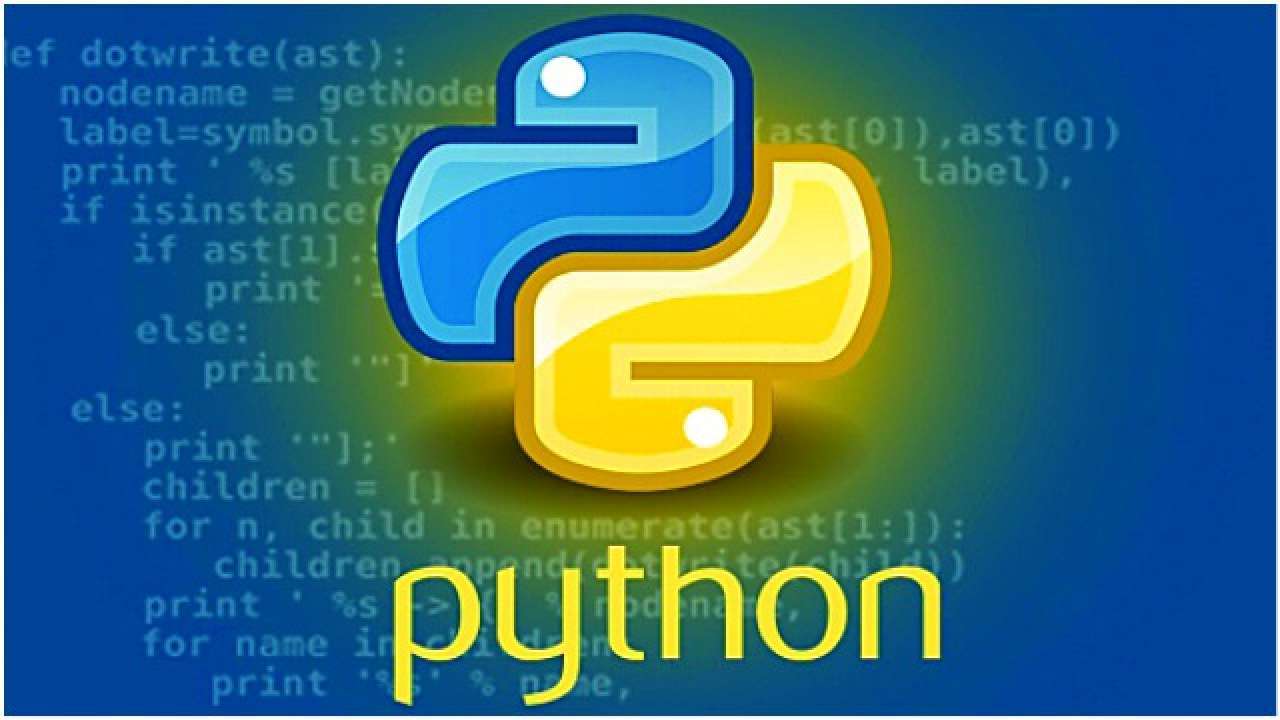
Introduction
Python is an interpreted, object-oriented, high-level programming language with dynamic semantics.
Its high-level built in data structures, combined with dynamic typing and dynamic binding, make it very attractive for Rapid Application Development, as well as for use as a scripting or glue language to connect existing components together. Python's simple, easy to learn syntax emphasizes readability and therefore reduces the cost of program maintenance. Python supports modules and packages, which encourages program modularity and code reuse. The Python interpreter and the extensive standard library are available in source or binary form without charge for all major platforms, and can be freely distributed.
Python
Python is often compared to other interpreted languages such as Java, JavaScript, Perl, Tcl, or Smalltalk. Comparisons to C++, Common Lisp and Scheme can also be enlightening. In this section I will briefly compare Python to each of these languages.
These comparisons concentrate on language issues only. In practice, the choice of a programming language is often dictated by other real-world constraints such as cost, availability, training, and prior investment, or even emotional attachment. Since these aspects are highly variable, it seems a waste of time to consider them much for this comparison.
print("Hello World") // This is how you get output
name = [1,2,3,4,5,6,7] // This is how you declare lists
- Variables
- Functions
- Control Flow
- Strings
- Dictionaries
Variables
Global variables are in fact properties of the global object. In web pages the global object is window, so you can set and access global variables using the window.variable syntax.
Consequently, you can access global variables declared in one window or frame from another window or frame by specifying the window or frame name. For example, if a variable called phoneNumber is declared in a document, you can refer to this variable from an iframe as parent.phoneNumber.
name_Surname = "Mlamuli Dimba" // Declaring a variable
Scope
When you declare a variable outside of any function, it is called a global variable, because it is available to any other code in the current document.
When you declare a variable within a function, it is called a local variable, because it is available only within that function.
Variable = "easy like this" // This is a variable
Functions
Functions store instruction to reusable code.
A function is a block of code which only runs when it is called. You can pass data, known as parameters, into a function. A function can return data as a result.
funcname(): //declaring a function
print("Hello") // local variable
funcname() // this is how you call a function